Classifying Handwritten MNIST Images
Using the Prototype container to quickly create models.
MNIST: The "hello world" of deep learning
MNIST is a standard academic dataset of binary images of handwritten digits. In this tutorial, we will see how to use a few methods to quickly set up models to classify the images into their 0-9 digit labels:
- The Prototype container model to quickly create a feedforward multilayer perceptron model from basic layers.
- Transform this Prototype into a Model of our own.
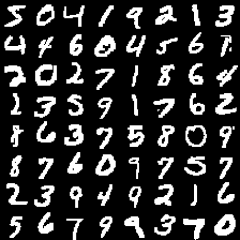
Some MNIST images.
Prototype: Quickly create models by adding layers (similar to Torch)
The opendeep.models.container.Prototype
class is a container for quickly assembling multiple layers together into a model. It is essentially a flexible list of Model objects, where you can add a single layer (model) at a time, or lists of models linked in complex ways.
To classify MNIST images with a multilayer perceptron, you only need the inputs, a hidden layer, and the output classification layer. Let's dive in and create a Prototype with these layers!
# imports
from opendeep.models.container import Prototype
from opendeep.models.single_layer.basic import BasicLayer, SoftmaxLayer
from opendeep.optimization.adadelta import AdaDelta
from opendeep.data.standard_datasets.image.mnist import MNIST
# create the MLP
mlp = Prototype()
mlp.add(BasicLayer(input_size=28*28, output_size=1000, activation='rectifier', noise='dropout'))
mlp.add(SoftmaxLayer(output_size=10))
# train the model with AdaDelta
trainer = AdaDelta(model=mlp, dataset=MNIST())
trainer.train()
Updated about 2 months ago