Installation/Getting Started
This page will help you get started with OpenDeep. You'll be up and running in a jiffy!
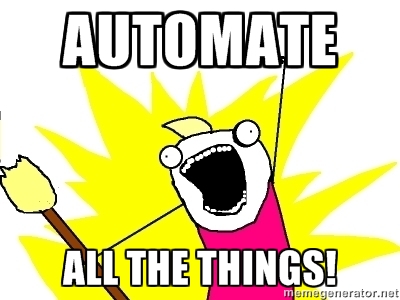
What is OpenDeep?
OpenDeep is a general purpose commercial and research grade deep learning library for Python built from the ground up in Theano that brings unprecedented flexibility for both industry data scientists and cutting-edge researchers.
You can train and use existing deep learning models as a black box implementation, combine multiple models create your own novel research, or write new models from scratch without worrying about the overhead!
OpenDeep is for you if you want:
- To not reinvent the wheel - instead you can pick and choose to use base implementations of datasets, optimization (training) algorithms, and popular models.
- Flexibility and extensibility to create novel models and optimization methods without having to think too hard about the framework - no one wants that cognitive load!
- Speed and stability of the Theano framework with effortless GPU integration for orders of magnitude speed improvements.
- Dependability for having implementations of popular and state-of-the-art algorithms.
Quick example usage: code an MLP in ~20 seconds
A Multilayer Perceptron is a simple feedforward network that has a classification layer. In this example, we can quickly construct a model container, add some hidden layers and an output layer, and train the network to classify images of handwritten digits (MNIST dataset).
from opendeep.models.container import Prototype
from opendeep.models.single_layer.basic import BasicLayer, SoftmaxLayer
from opendeep.optimization.adadelta import AdaDelta
from opendeep.data.standard_datasets.image.mnist import MNIST
mlp = Prototype()
mlp.add(BasicLayer(input_size=28*28, output_size=512, activation='rectifier', noise='dropout'))
mlp.add(BasicLayer(output_size=512, activation='rectifier', noise='dropout'))
mlp.add(SoftmaxLayer(output_size=10))
trainer = AdaDelta(model=mlp, dataset=MNIST())
trainer.train()
Installation
Dependencies
-
Theano: Theano and its dependencies are required to use OpenDeep. You need to install the bleeding-edge version, which has installation instructions here.
-
For GPU integration with Theano, you also need the latest CUDA drivers. Here are instructions for setting up Theano for the GPU. If you prefer to use a server on Amazon Web Services, here are instructions for setting up an EC2 server with Theano.
-
CuDNN (optional): for a fast convolutional net support from Nvidia. You will want to move the files to Theano's directory like the instructions say here: Theano cuDNN integration.
-
-
Pillow (PIL): image manipulation functionality.
-
PyYAML (optional): used for YAML parsing of config files.
-
Bokeh (optional): if you want live charting/plotting of values during training or testing. Make sure you can use the bokeh-server command.
Install from source
Because OpenDeep is still in alpha, you have to install via setup.py. Here are the steps to install:
- Navigate to your desired installation directory and download the github repository like so:
git clone https://github.com/vitruvianscience/opendeep.git
- Navigate to the top-level folder (should be named OpenDeep and contain the file setup.py) and run setup.py with develop mode like so:
cd OpenDeep
python setup.py develop
Using python setup.py develop
instead of the normal python setup.py install
allows you to update the repository files by pulling from git and have the whole package update! No need to reinstall.
That's it! Now you should be able to import opendeep into python modules.
Next Steps
Check out some Tutorials in the navigation bar on the left.
To learn how to use existing models, check out Tutorial: First Steps.
A good place to start writing models is Tutorial: Your First Model (DAE), where you will learn how to create a new model from scratch.
Updated less than a minute ago